December 15, 2023
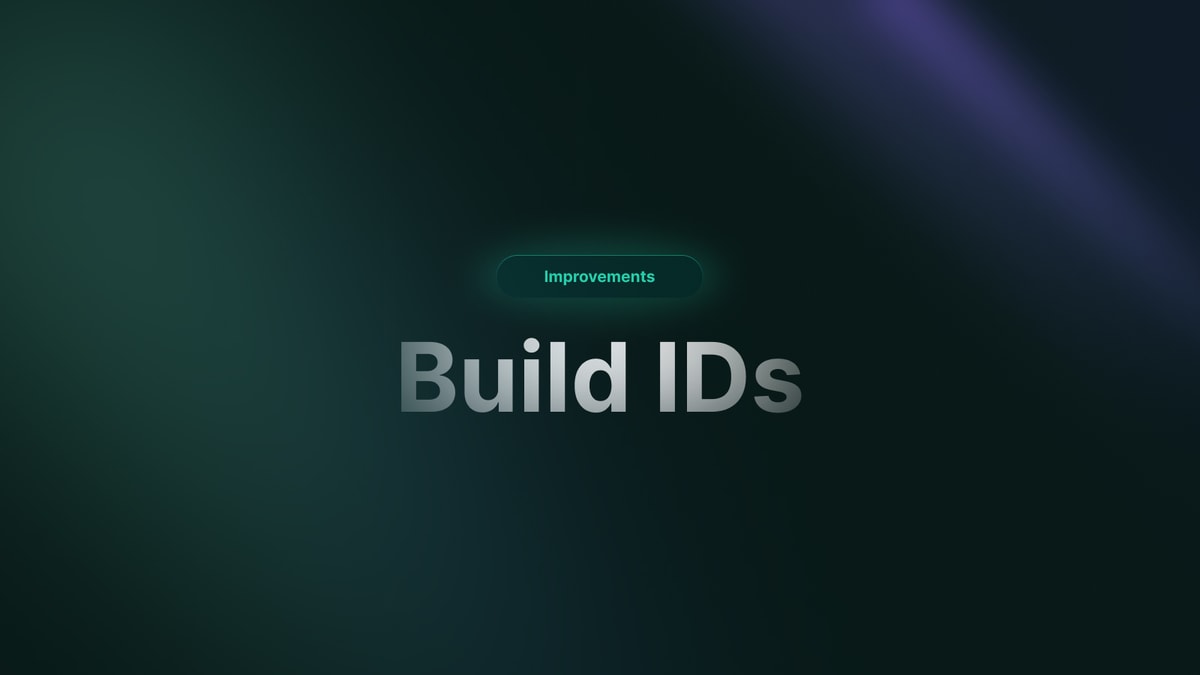
Build IDs
Another week in the bag. This week's wrap will be short and sweet.Associating bugs with build IDsThis week we added `buildId` to the captures.
The build ID is something that gets generated at build time. It is used to push artifacts to the API, and will be bound to all bug captures stemming from that build.
You can use the build ID to for instance associate each build with a Git commit hash, allowing you to understand the state of your codebase when going through bug-reports.
Below is an example of how you can use the `generateBuildId` to associate captures with a Git commit hash with Vercel.
import { defineFlytrapConfig } from "useflytrap"export default defineFlytrapConfig({generateBuildId: () => {return process.env.VERCEL_GIT_COMMIT_SHA ?? crypto.randomUUID()}})
You can start using the `buildId` by upgrading to useflytrap@0.9.2
We would love to hear your feedback / suggestions on Twitter or Discord!
Improvements and fixes
December 4, 2023
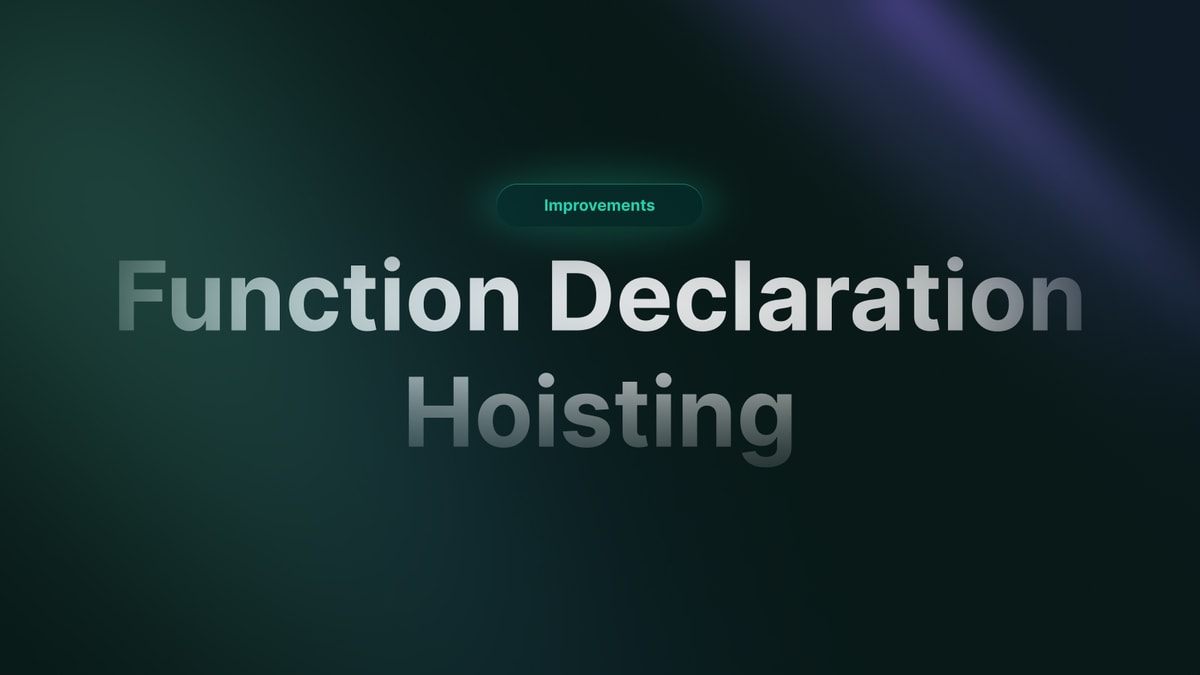
Function Declaration Hoisting
Phew! Slush is in the bag. Last week was hectic, eventful and slightly tiring. We witnessed among other things, a person pull a pint of beer with the power of thought.π€― But now we're back to shipping!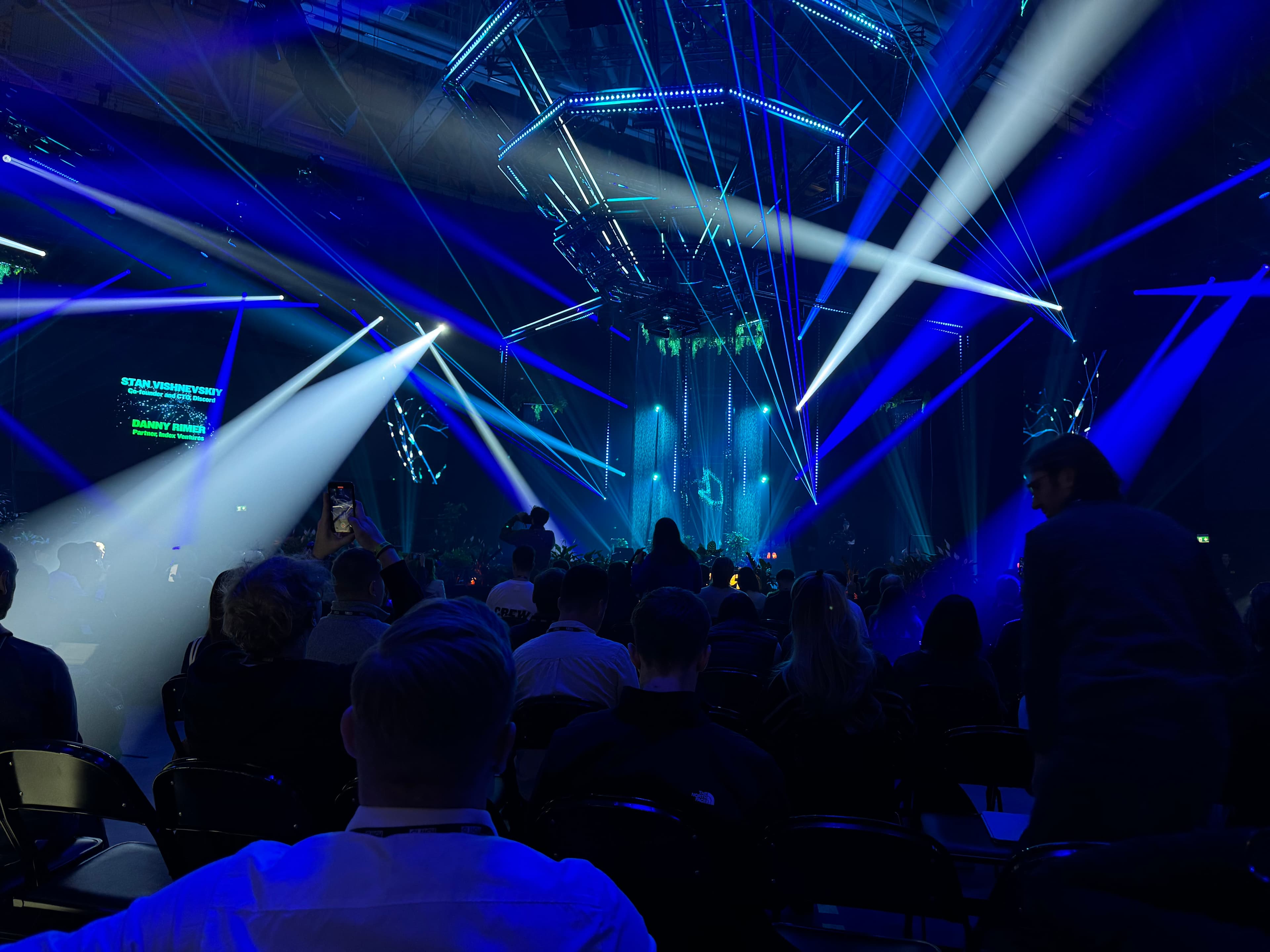
In cases where you used function declarations before they were declared in code, the code would not run after transforming with Flytrap. Because of this, we shipped an improvement that automatically hoists all function declarations to the top of their respective scopes, while respecting things such as imports and directives.
You can use the function declaration hoisting by upgrading to useflytrap@0.9
We would love to hear your feedback / suggestions on Twitter or Discord!
Improvements and fixes
November 28, 2023
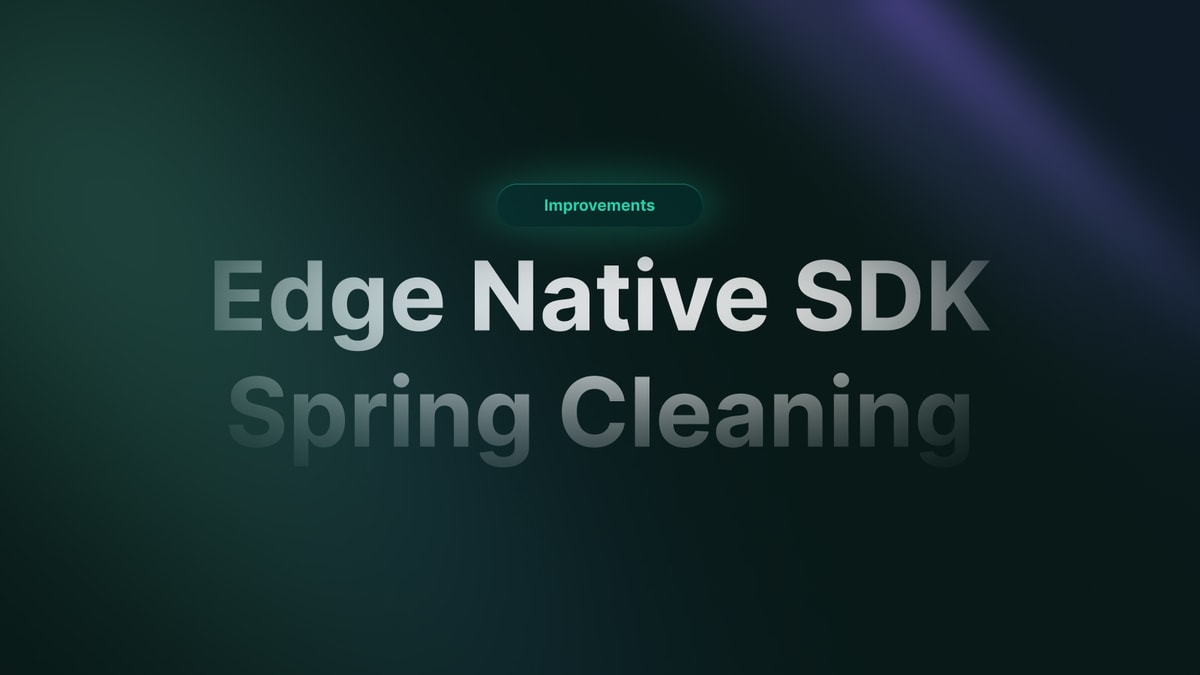
Edge Native SDK & Spring Cleaning
Another week in the bag. As we set our sights on Slush this upcoming week, here's a quick recap what we shipped last week.Edge Native SDKThe Flytrap SDK is now "edge" / "browser" -native, whatever you want to call it, hurray!π What we mean by that is that we only use JavaScript Standard APIs for the whole SDK, starting from useflytrap@0.8.The APIs we use are supported by most edge runtimes (eg. Vercel Edge Functions, Cloudflare Workers, Deno Deploy), so you can now use Flytrap to get detailed bug-captures even from your edge functions!π€©
The code that previously required non-browser code such as require() is no longer included in the core Flytrap SDK, and only gets dynamically included when you're in mode === 'replay'. Meaning, no changes required by you!
Spring CleaningWe know, we know⦠It's not spring yet, but it might aswell be, with the amount of spring cleaning we have been doing on the codebase the last week!
In just one commit alone, we got rid of 500 lines of code, while actually improving the simplicity, error handling & reusability of the code-base.
We rewrote almost every part of our code-base with Rust inspired error handling with the libraries ts-results & human-logs (by yours trulyπ)!
We will release a more in-depth blog post about how we think about error handling, how it can improve a product massively and how we structure our code with an open-source library we wrote to handle errors super intuitively.
We would love to hear your feedback / suggestions on Twitter or Discord!
Improvements and fixes
November 20, 2023
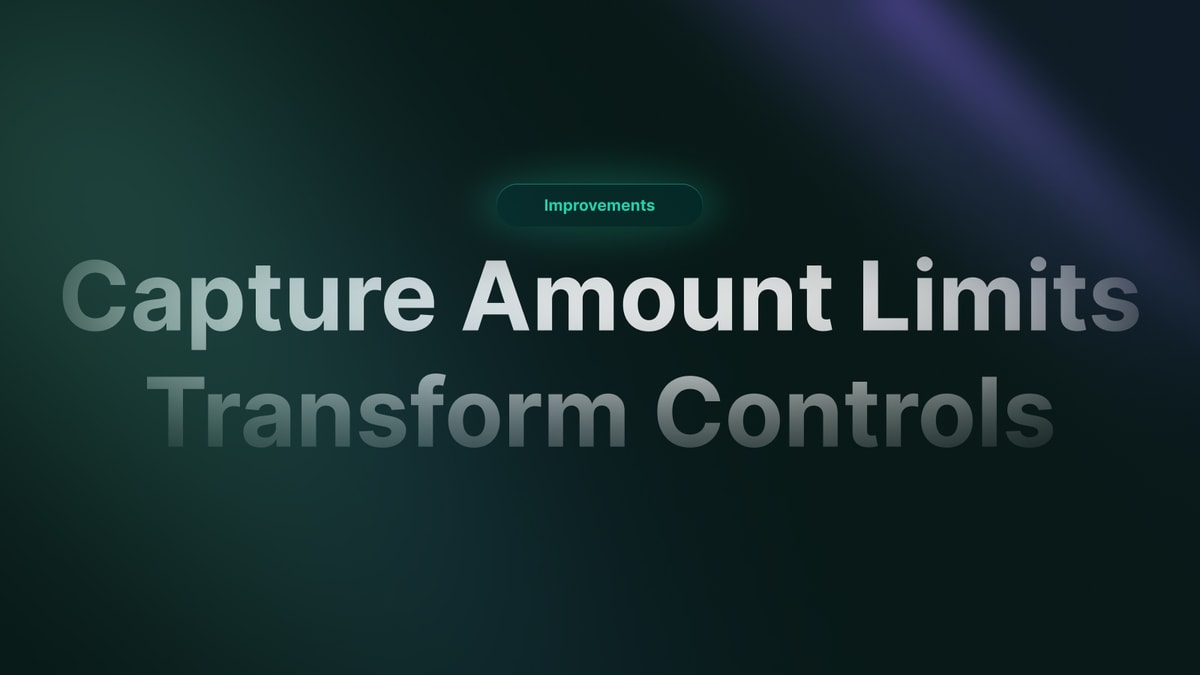
Capture Amount Limit, Advanced Transformation Controls
"Don't Stop 'til You Ship Enough" - Michael JacksonAnd we wont. Last week we shipped many features once again improving the Flytrap SDK further. π€©Capture Amount ConfigurabilityOne of the greatest benefits that Flytrap provides is the data of every function call and function leading up to end-user bugs. However, while having this data is super helpful for finding the root cause of bugs, often times the data we need to find the root-cause happens to be in one of the most recent source-files that executed.
Because of this, we added the possibility to configure the amount of context that is sent to Flytrap, whenever end-users encounter bugs. We implemented this in an intuitive way, where you can define it using an amount of files (eg. "5 files", meaning that the context from the last 5 files will be sent) or raw size of capture (eg. "1 mb", meaning that the capture will be a maximum of 1 megabyte).
This allows you to save on usage by defining the amount of data you want Flytrap to capture. To configure the capture amount, set the `captureAmountLimit` flag in your Flytrap config:
import { defineFlytrapConfig } from "useflytrap"export default defineFlytrapConfig({projectId: 'your-project-id',publicApiKey: '*****',secretApiKey: '*****',privateKey: '*****',packageIgnores: ['next/font', 'zod'],captureAmountLimit: '5 files'})
You can disable the transformation of `function-declaration`, `function-expression`, `arrow-function` or `call-expression`.
For instance, if you use function declarations in code, before their definition, you can now disable the transformation of them, to not risk running into unexpected runtime errors. (Then you won't capture the context either unfortunately, which is why we recommend that you instead move function declarations so that they get defined before they're used in their lexical scope.)
To use it, simply modifify the `transformOptions.disableTransformation` to include one of the below values.
import { defineFlytrapConfig } from "useflytrap"export default defineFlytrapConfig({projectId: 'your-project-id',publicApiKey: '*****',secretApiKey: '*****',privateKey: '*****',packageIgnores: ['next/font', 'zod'],transformOptions: {disableTransformation: ['function-declaration', 'arrow-function', 'function-expression', 'call-expression']}})
CommonJS Support for Generated CodeAlso a small but welcome addition was the support for CommonJS in the code that Flytrap generates. This will benefit people who are still waiting to make the jump to ES Modules. To use CommonJS, simply configure it in the Flytrap config:
import { defineFlytrapConfig } from "useflytrap"export default defineFlytrapConfig({projectId: 'your-project-id',publicApiKey: '*****',secretApiKey: '*****',privateKey: '*****',packageIgnores: ['next/font', 'zod'],transformOptions: {importFormat: 'commonjs'}})
Note
The setting for `importFormat` will be overriden for files that contain the extension .cjs or .mjs
Improvements and fixes
November 11, 2023
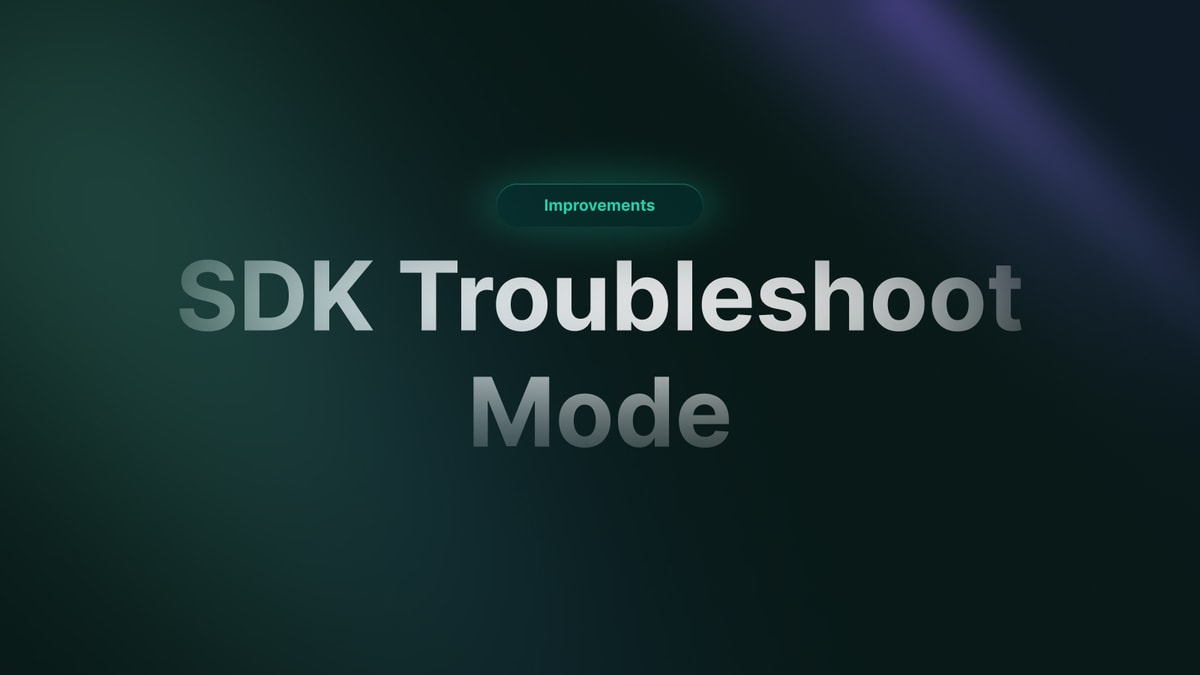
Flytrap SDK Troubleshooting Mode
This week's wrap will be short and sweet. Keeping up with the theme from last week, we focused on doing some improvements to the Flytrap SDK itself.Troubleshooting ModeIf something isn't working as it should with the Flytrap SDK, you can use troubleshooting mode to understand why. Troubleshooting mode will hopefully make it easier to understand if some problems occur, and help us understand and support our users better.
To start using the troubleshooting mode, install Flytrap with with the tag useflytrap@next, and update the mode to 'troubleshoot' in your Flytrap config:
import { defineFlytrapConfig } from "useflytrap"export default defineFlytrapConfig({projectId: 'your-project-id',publicApiKey: '*****',secretApiKey: '*****',privateKey: '*****',mode: 'troubleshoot',packageIgnores: ['next/font', 'zod']})
November 3, 2023
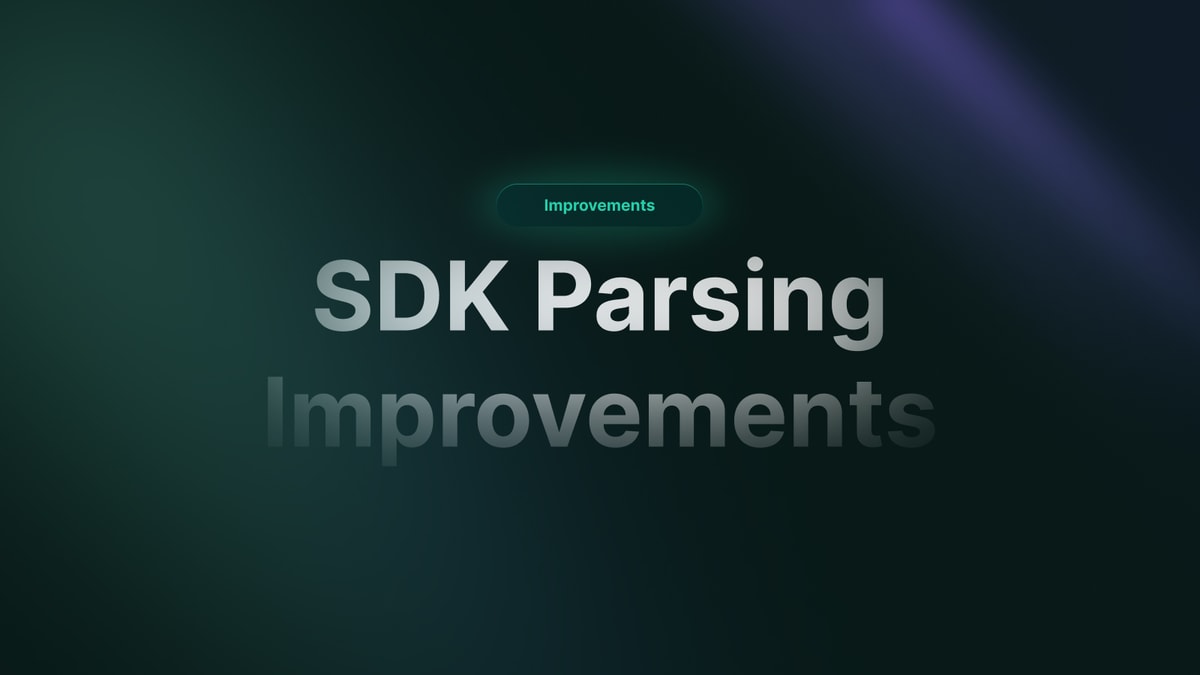
Flytrap SDK Parsing Improvements
This week the team's focus has been on doing some improvements to the Flytrap SDK itself, along with some improvements to our capture APIs.Improved ParsingWhen building a product where you have to be able to transform all JavaScript code that people have in their unique codebases, problems arise quite quickly. Because of that, we realised that we need to allow users to configure the parse options to match their unique codebases. You can now set the parser options with the babel.parserOptions key in the Flytrap configuration file.
Also, the parser errors have gotten much more human-friendly, and are inspired by Rust's super helpful error messages, check them out below!
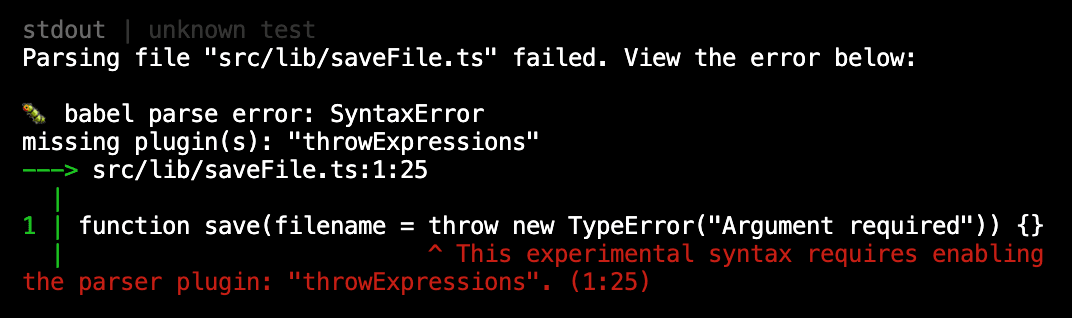
However, with time, Vercel's platform limits started limiting Flytrap's ability to capture certain bugs. Due to this, we have now deployed our custom Capture API at https://api.useflytrap.com
Using this API allows us to save captures without any restrictions. To use the new Capture API, configure the apiBase option in your flytrap.config.ts file to https://api.useflytrap.com
Improvements and fixes
October 19, 2023
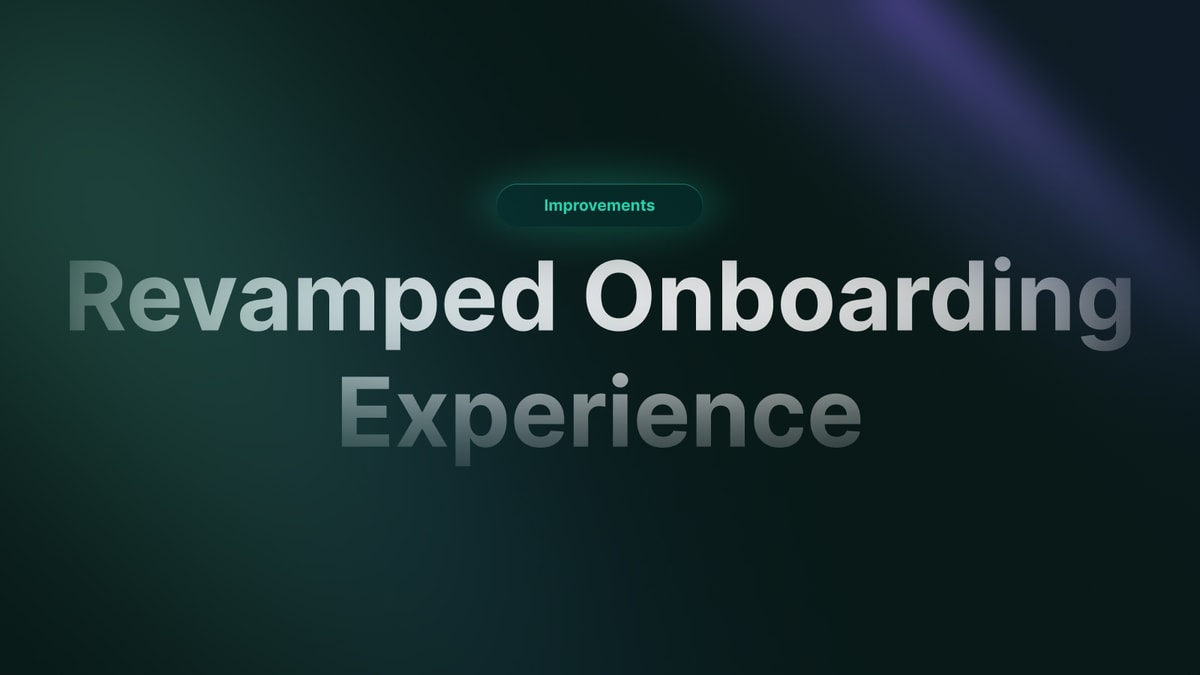
Revamped Onboarding Experience
Another Friβ¦ Thursday, another changelog. That's right, a changelog on a Thursday, we're living through strange times.πΈThis week we're a bit lighter on the changes to the product, however we still shipped a change that will improve the overall experience with the product, namely **drumroll**
Revamped Onboarding Experience πͺThe new onboarding experience is designed to take you from signup to your "Heureka!"-moment β seeing your first bug capture on the Flytrap dashboard. We made the onboarding such that it tells you exactly what to do, and everything should work with just copy-paste. Feedback on the onboarding is much appreicated!π
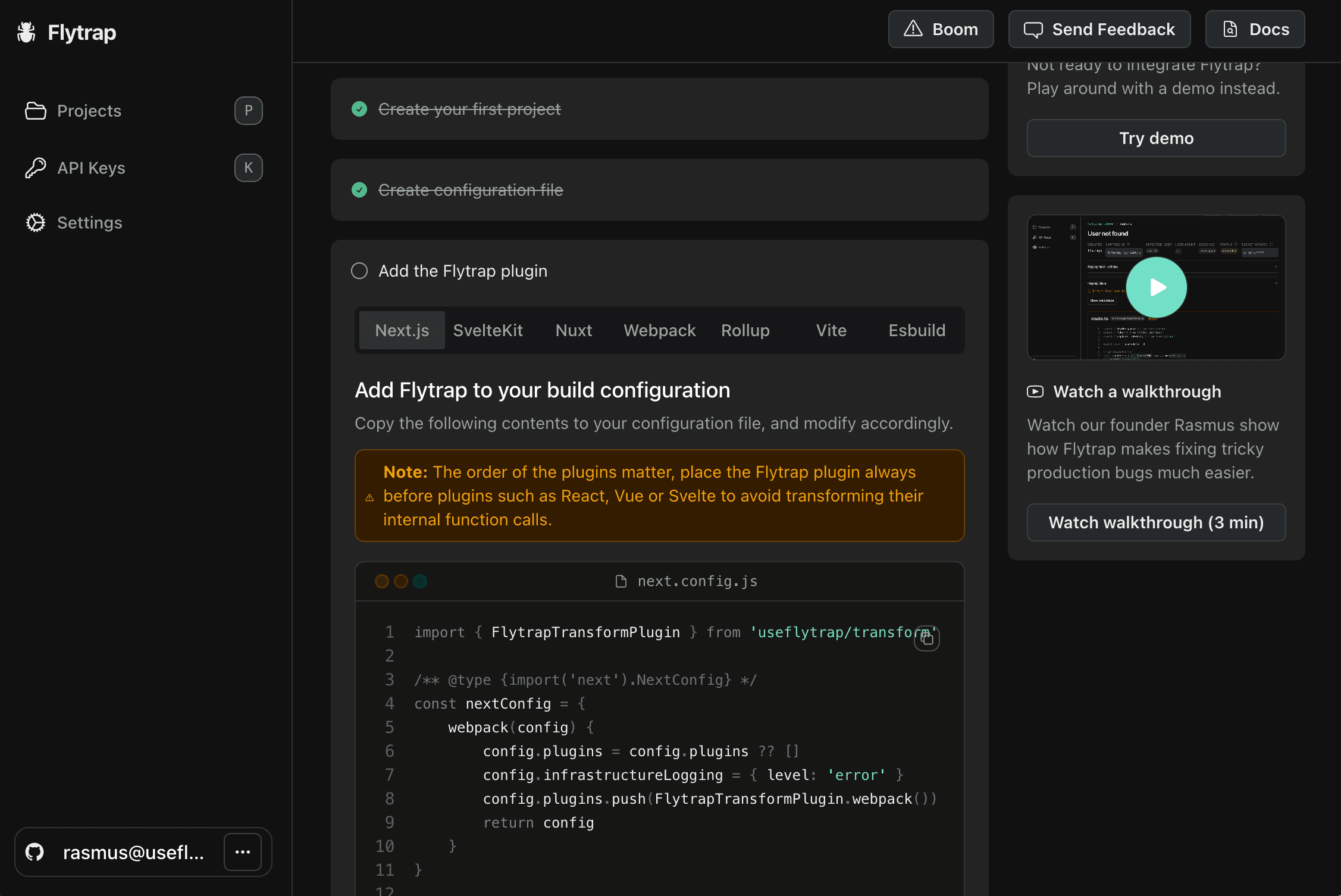
We also decided to build an open-source onboarding library to make building smooth, persistent onboarding flows simple as possible. We're going to release that in the coming weeks so stay tuned!π€©
Give the new onboarding flow a try and let us know your feedback / suggestions on Twitter or Discord!
Improvements and fixes
September 15, 2023
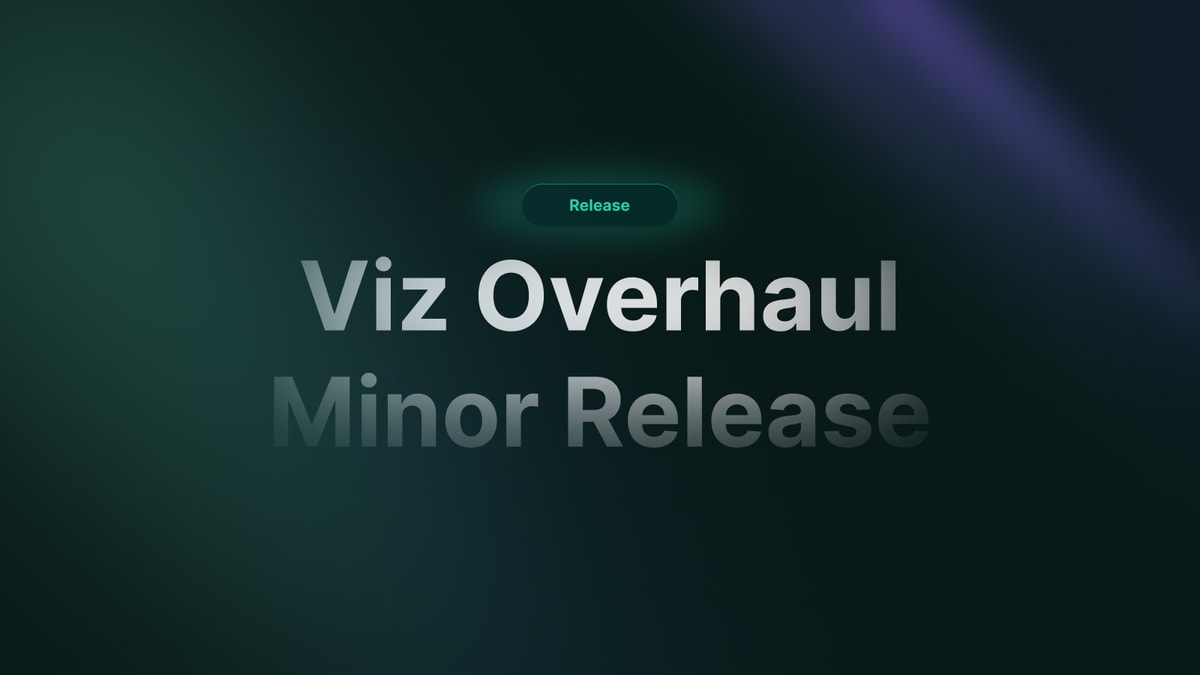
Major Bug Visualization Improvements, Minor Release
Schools are starting, summer is rounding to an end. But unlike the summer, the changelogs will continue. πThis week we're more excited than ever about what we're releasing. This changelog is packed with major and minor changes to share. Let's get to it!Major Bug Visualization Improvements πToday we're SUPER excited π₯³ to announce the final form (or is it?) of the bug visualization experience. Our vision was always to be able to view the bugs and data leading to those bugs right in our source-code, and today, that day is here.
Features:
β View bugs right in your source-code, click on functions and function calls to see their inputs and outputs. With Flytrap, you really need no manual logging setup, because with Flytrap, EVERYTHING is logged.
β The hoverable content is color-coded, allowing you to quickly see which functions ran fine and without errors, and which had errors.
To see how the new improved bug visualization looks like, check out the video below or try it out for yourself here.
To use the new improved visualization, upgrade your useflytrap to v0.6, and build your project. You can now head to the Flytrap dashboard and see all your captures with much more clarity.Version 0.6 ReleaseStarting from useflytrap@0.6, the new artifacts will be used and the use of the old artifacts will be deprecated. If you're using the old artifacts, you will get a notice to upgrade.
To start using the new bug capturing experience that v0.6 enables, simply upgrade your useflytrap package, and build your code. Now all your bugs will be able to be viewed as if they were running inside your code files.
Give the new release a try and let us know your feedback / suggestions on Twitter or Discord!
Improvements and fixes
August 28, 2023
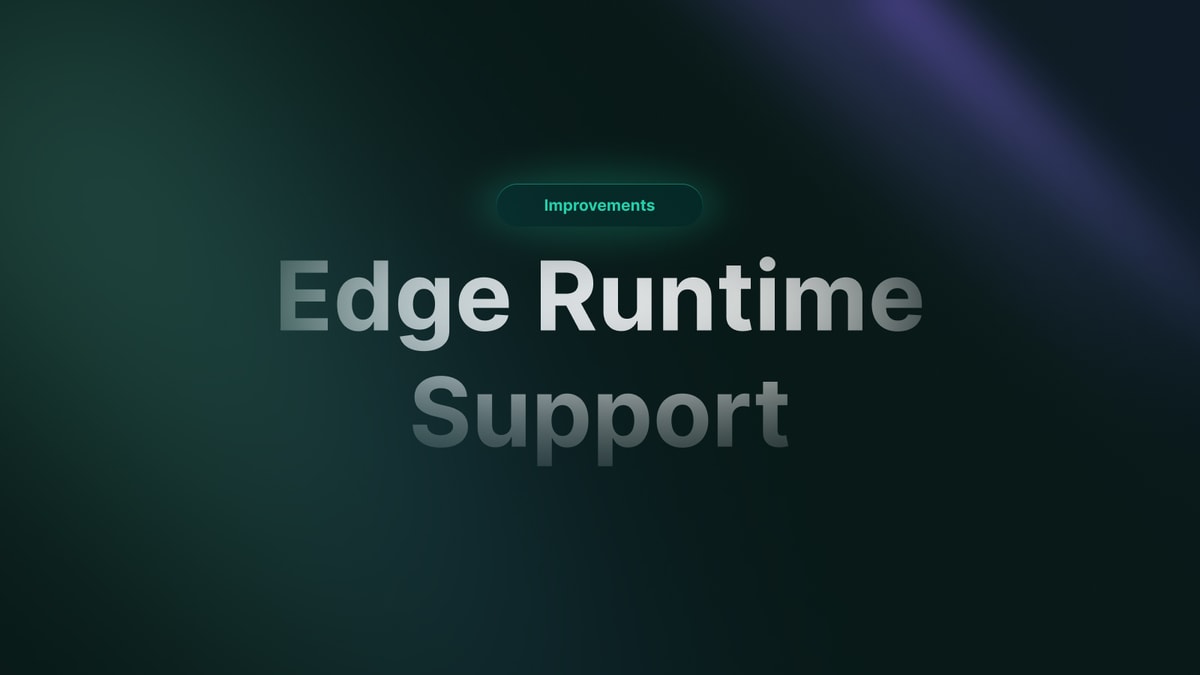
Support for Edge Runtimes
As per our focus of being the go to debugging tool for modern web development (both back- and front-end), we set aside a little time to make the experience even smoother for our friends living on the edge (pun intended), which is why we shipped support for edge runtimes.Edge Runtimes SupportBy default, the useflytrap has always been isomorphic during runtime, but since it is not enough for in some environments / setups (like SvelteKit), we decided to ship a version of Flytrap that only ever uses & imports browser & Web APIs.If you want to use Flytrap in an edge environment, starting from v0.5.3 you can add the browser key in your Flytrap config file, as shown below.
import { defineFlytrapConfig } from "useflytrap"export default defineFlytrapConfig({projectId: 'project-id',publicApiKey: 'blah blah',secretApiKey: '*****',privateKey: '*****',mode: 'capture',browser: true})
August 18, 2023
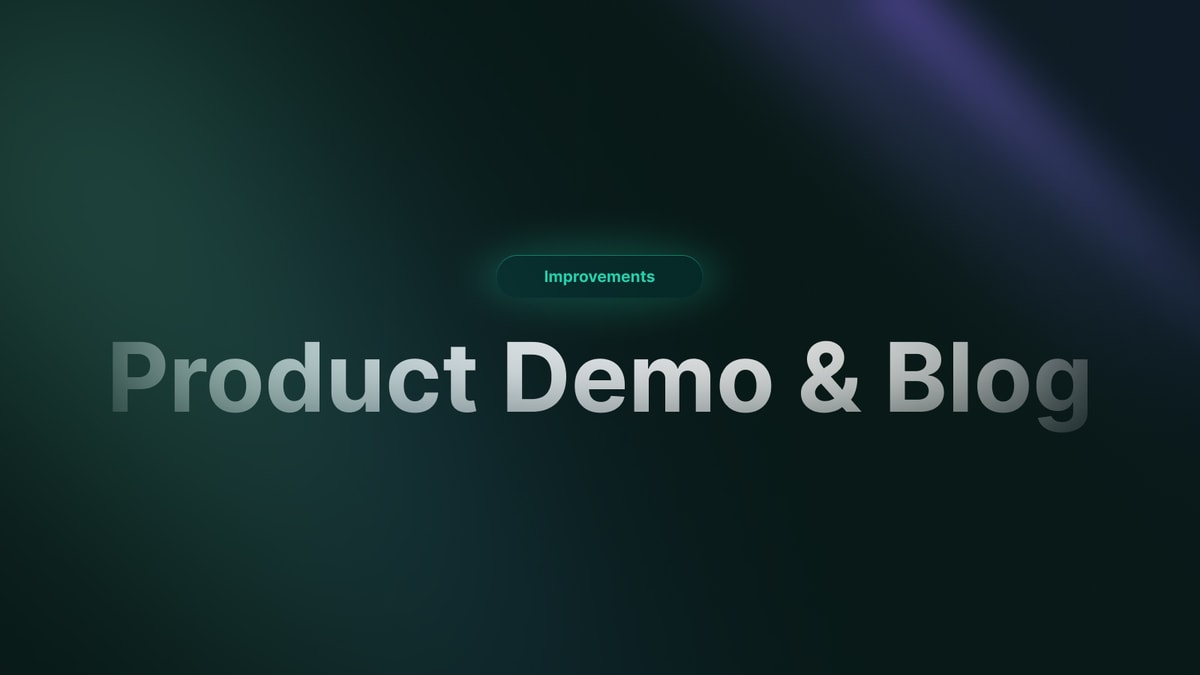
Publicly Available Product Demo, Releasing Flytrap Blog
Long time no changelog! The Flytrap team has been diligently working on a lot of moving operational things behind the curtains, but now the changelog is back!Publicly Available Product DemoThere's nothing that developers love more than being able to see and interact with a tool before putting down the time to integrate the tool into their own codebase. Especially when it comes to a tool like Flytrap, where you have to see how good it is to believe itπ€©Because of this, we have made a publicly available product demo, where anyone can go and play around with the product and see the value.
Try it out for yourself here.
Flytrap BlogWe're also releasing our blog. Our blog will contain writings about how we do engineering at Flytrap, product guides and how-to's about using Flytrap to fix production bugs fast as lightning and general posts focusing on creating software in a way that bugs are easy to fix.
July 22, 2023
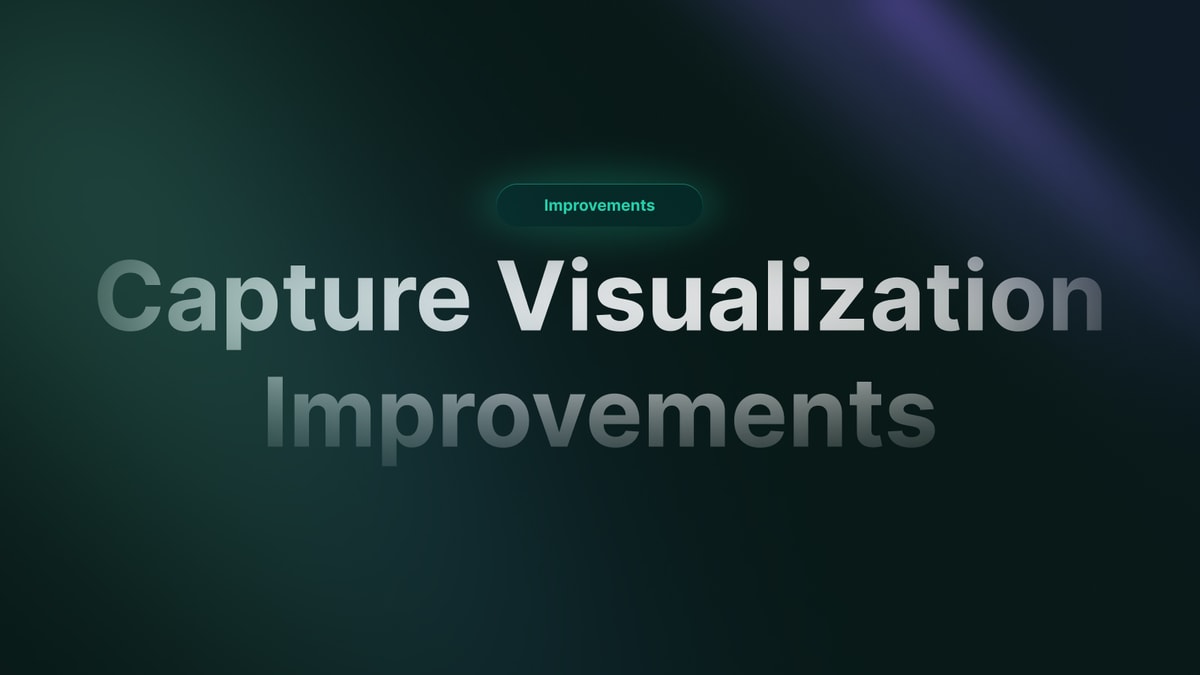
Capture Visualization Improvements, Artifacts Versioning
Another week in the bag, you know what that means! Time for yet another changelog! The last speek was spent mainly focusing on some really nice Quality-of-Life improvements, before we move on to the larger moving rocks the coming weeks.Capture Visualization ImprovementsWe looove when we can make the captures that land in the Flytrap much more readable and easier to understand, to make it even easier for developers to find the root of the problem. Today we are releasing quite a nice Quality-of-Life improvement to the capture experience, check it out below!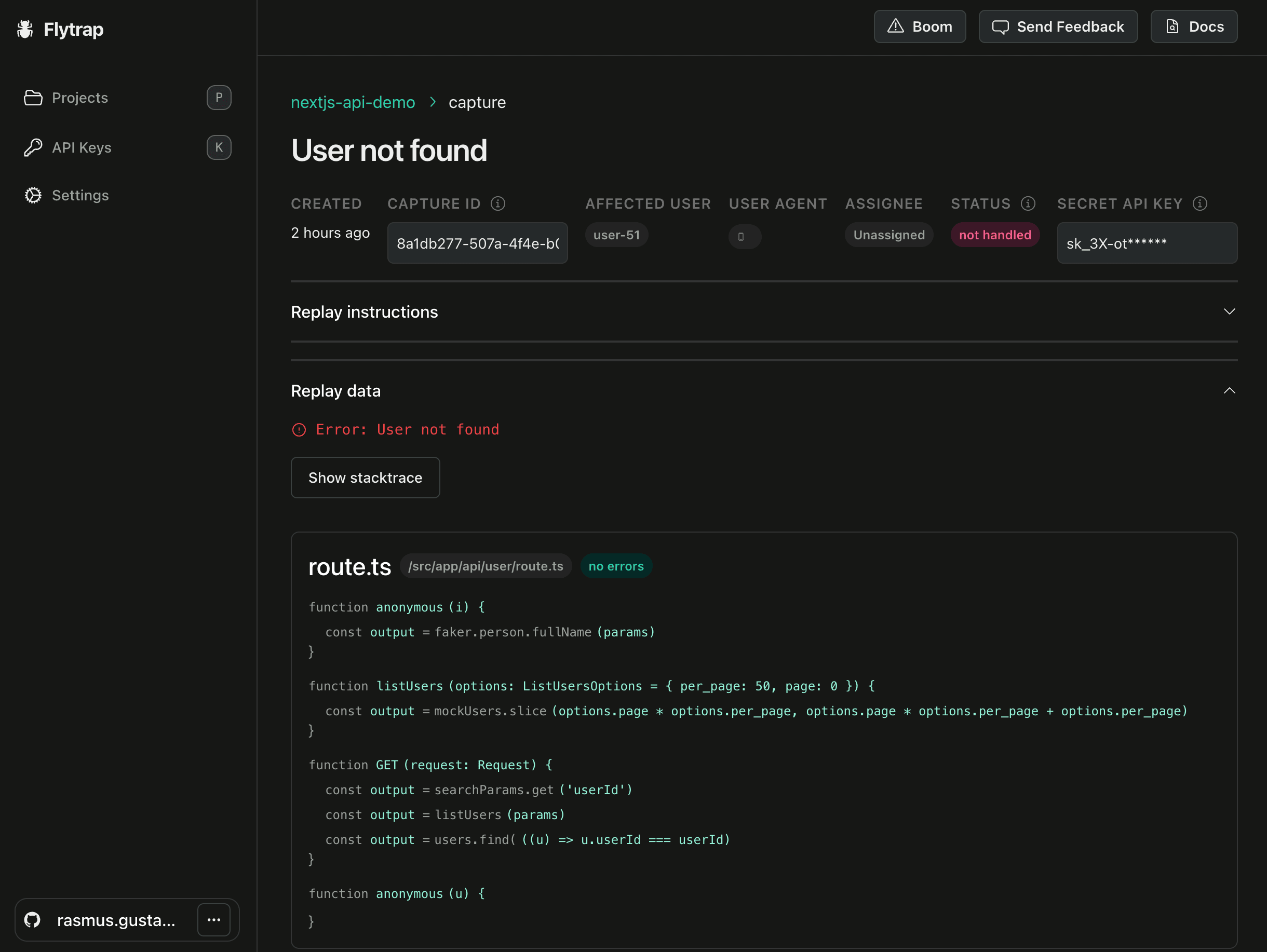
Artifacts VersioningAnother thing we shipped this week is versioning for Artifacts. While this is more of a behind-the-scenes type of improvement, it does also improve your user-experience! Basically this update allows your captures to match the structure of your codebase, even as it changes, so older captures will still look perfect, even though you have made changes to your codebase!π
Don't be afraid to reach out with your ideas and feedback!
Improvements and fixes
July 5, 2023
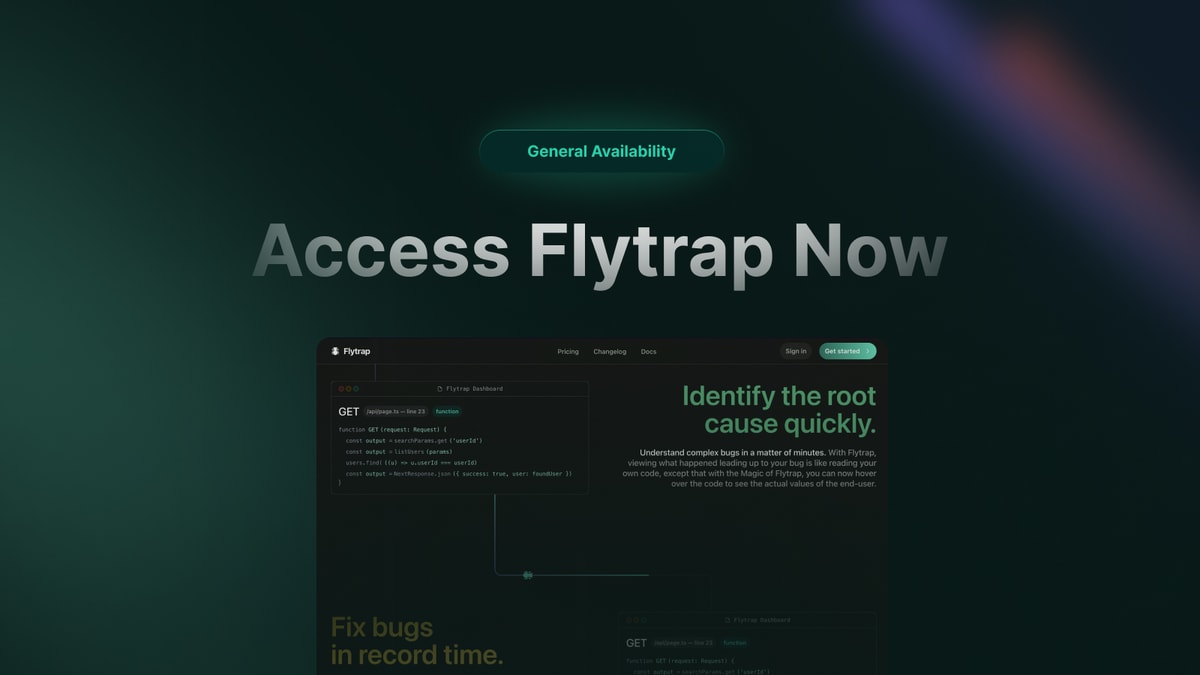
Flytrap General Availability Launch
Flytrap is now publicly available! We are thrilled to announce this significant milestone after months of extensive hard work and dedication from our team. We have polished various minor issues and prepared all the aspects of Flytrap for a broader audience, empowering developers to debug production bugs with speed and efficiency.PricingWe added a Free tier to the pricing, which allows you to use Flytrap for personal & smaller projects.Don't be afraid to reach out with your ideas and feedback!
Improvements and fixes
June 26, 2023
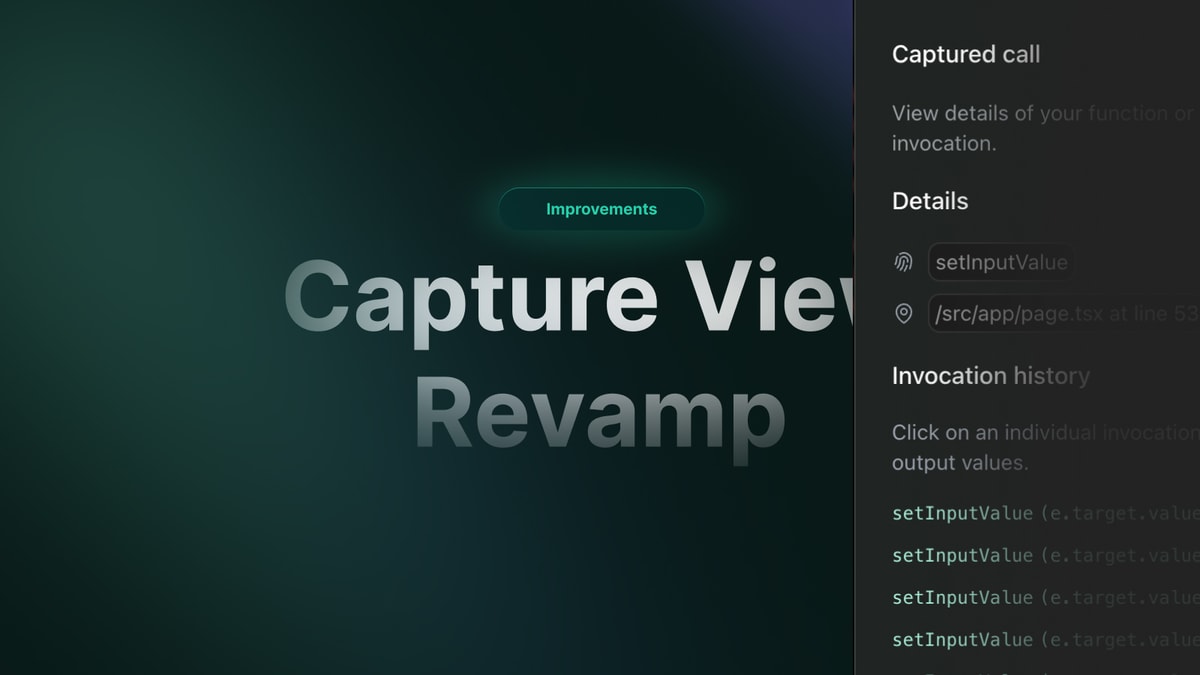
Improved Capture Viewing & Capture Ignores
This changelog marks the last changelog during the private beta! We're more excited than ever to finally launch Flytrap to a wider audience. The one last thing we decided to ship before General Availability was an even further improved capture viewing experience, making it so much easier to pinpoint the source of the bug, and quickly fix it. With that being said; here's what we shipped last week:Improved Capture ViewingThis week, we gave the capture viewing experience a big improvement, with our revamped capture view. Now, it only shows the function calls once, and you can view the history of function call invocations by clicking on the capture. This brings us closer to our long term goal of having it completely match your code (but thats a story for a later changelogπ).
With the improved capture viewing experience, it's much easier to understand what happened leading up to the error, by examining the invocation history of functions and function calls.Capture IgnoresA big part of our core beliefs is that many current tools are like The Boy Who Cried Wolf, often sending error reports from simple failed network requests et cetera. We want to be the opposite. We want every capture to land in the Flytrap to actually be a meaningful signal that there exists a bug in the code. To achieve this, we added the option to ignore certain captures based on some condition. With Capture Ignores, you can now ignore based on simple string matching, Regular Expressions or a function, which allows you great flexibility.
For example, to ignore captures which name contains "UNAUTHORIZED". You can learn more on our configuration docs page.
import { defineFlytrapConfig } from "useflytrap"export default defineFlytrapConfig({projectId: 'project-id',publicApiKey: 'blah blah',secretApiKey: '*****',privateKey: '*****',mode: 'capture',captureIgnores: ['UNAUTHORIZED']})
We're super excited about the coming weeks, and look forward to General Availability!
Improvements and fixes
June 16, 2023
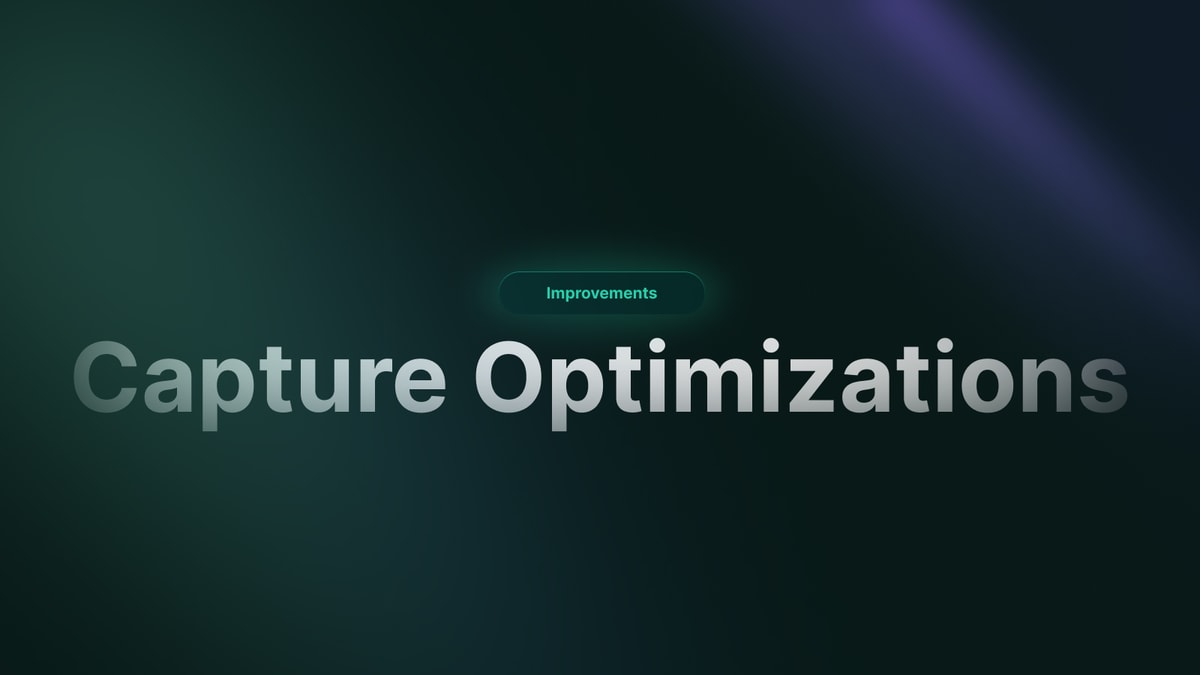
Capture & Artifacts Optimizations
This week the team has continued with the focus on foundational work and pushing Flytrap towards General Availability. One of the key foundational things that needed to change before reaching a stable release was an improved structure for captures. So with that in mind, here's what we shipped last week:Capture OptimizationsThis week, we optimized the way that capture data is structured when being sent to the Flytrap API. We did a major refactor of the way things were structured, which allowed us to get up to a 75% decrease in capture size. This means that you will be able to fit far more captures into your usage quota than before!
To take the new optimizations into use, upgrade your useflytrap package to version 0.2.1
Improvements and fixes
June 12, 2023
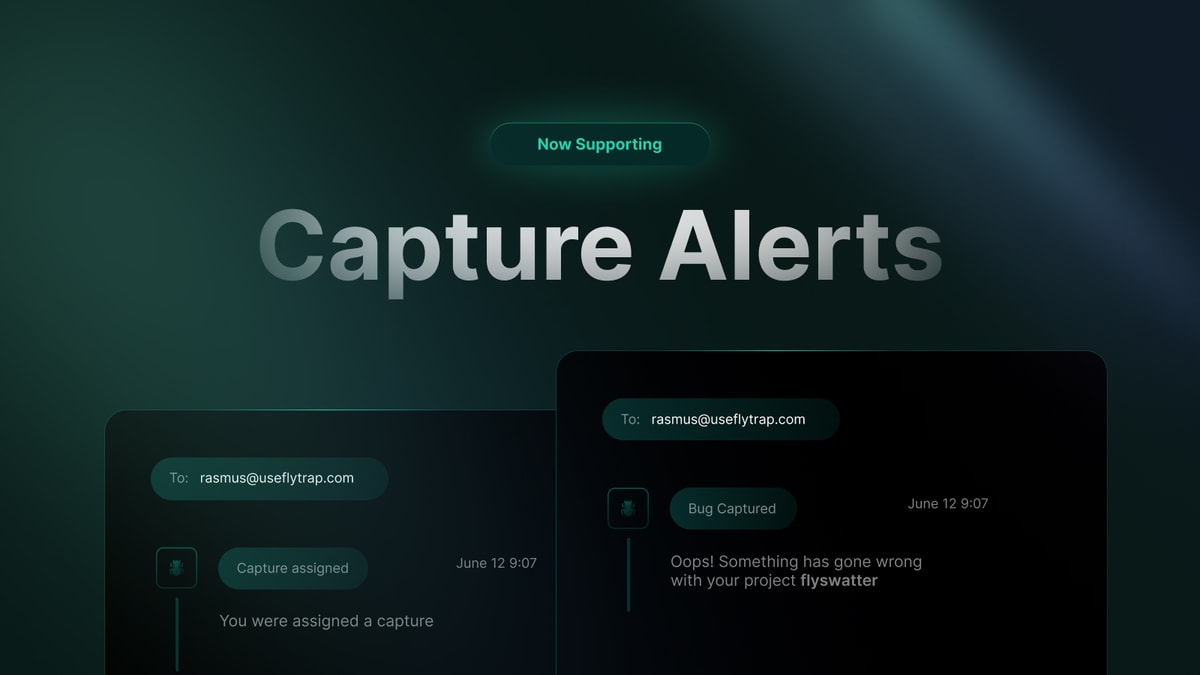
Capture Email Alerts, Assign Alerts
This week the team shifted focus for a bit, focusing on the "foundational work" instead of new features. This week marks the start of the sprint towards General Availability. We're more excited than ever to put Flytrap in the hands of even more people in the coming weeks! But even so, we couldn't leave you empty handed, so here's what we shipped last week:Capture & Assign Email AlertsOne of the most important thing about a tool like Flytrap, is that you're immediately notified when something goes wrong. To this end, we added email notifications whenever a bug is captured.
You can change your email preferences in the settings page. You can now decide to get notifications when captures are created, or when you're assigned to a capture. You can also choose to unsubscribe from the changelog updates (although we're sure no one will want to do so π).
Improvements and fixes
June 5, 2023
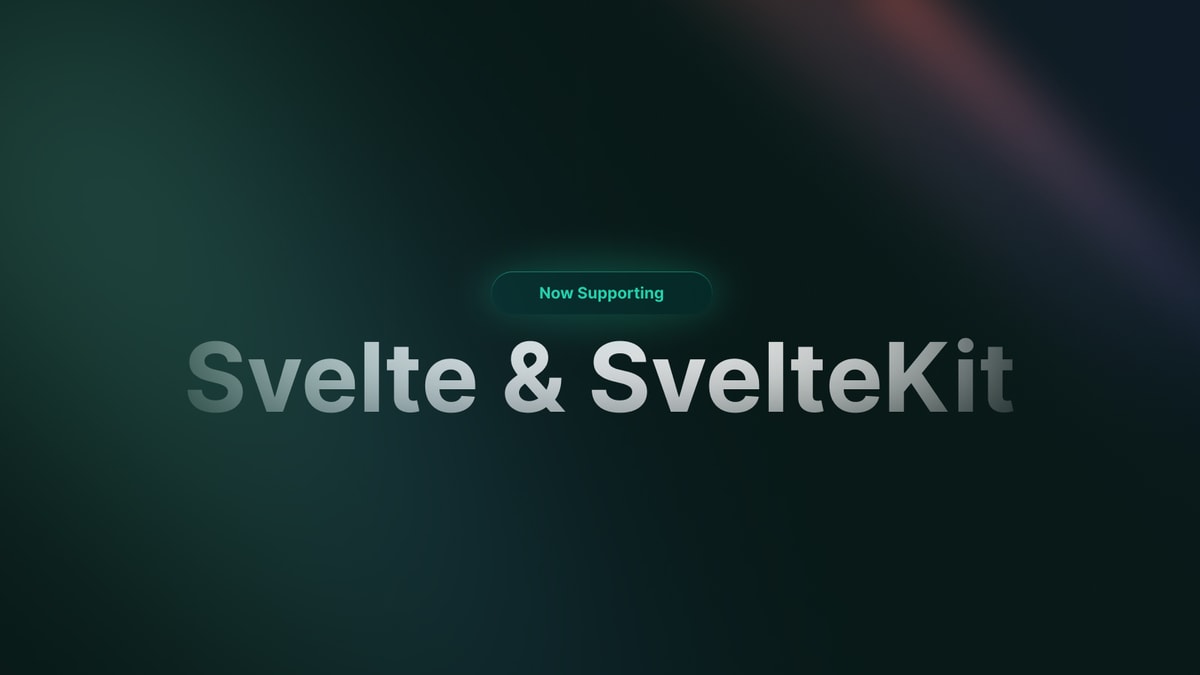
Svelte & SvelteKit Support, Filtering Captures & Assigning Members
A monday changelog for a change, how refreshing! Continuing from last week's theme of expanding the horizons of Flytrap, this week we are releasing support for Svelte & SvelteKit. We also shipped many Quality-of-Life improvements that we as a team had long been longing for!Here's what we shipped last week:
Svelte & SvelteKit supportNow you can integrate your favorite bugcatching tool to your Svelte & SvelteKit projects! Read our installation Docs for SvelteKit or check out our example project here.
Note, that while capturing works like butter, replay for Svelte, SvelteKit (& Vue and Nuxt) are still being worked on, as these frameworks don't explicitly handle state the way that React does, so make sure to stay tuned!Filtering Captures / Revamped Captures ListThis one is a huge Quality-of-Life improvement for us when we are internally using Flytrap, and as the amount of captures for projects on Flytrap grow, it was time to be implemented.
With the new capture list view, we're able to easily change the status, priority of captures, delete one or many captures super easily. We're also able to search for captures using the name of the capture and affected user.
Assigning MembersOn the Quality-of-Life front, we can now also assign team members to captures to help with managing workloads when fixing bugs.
Improvements and fixes
May 26, 2023
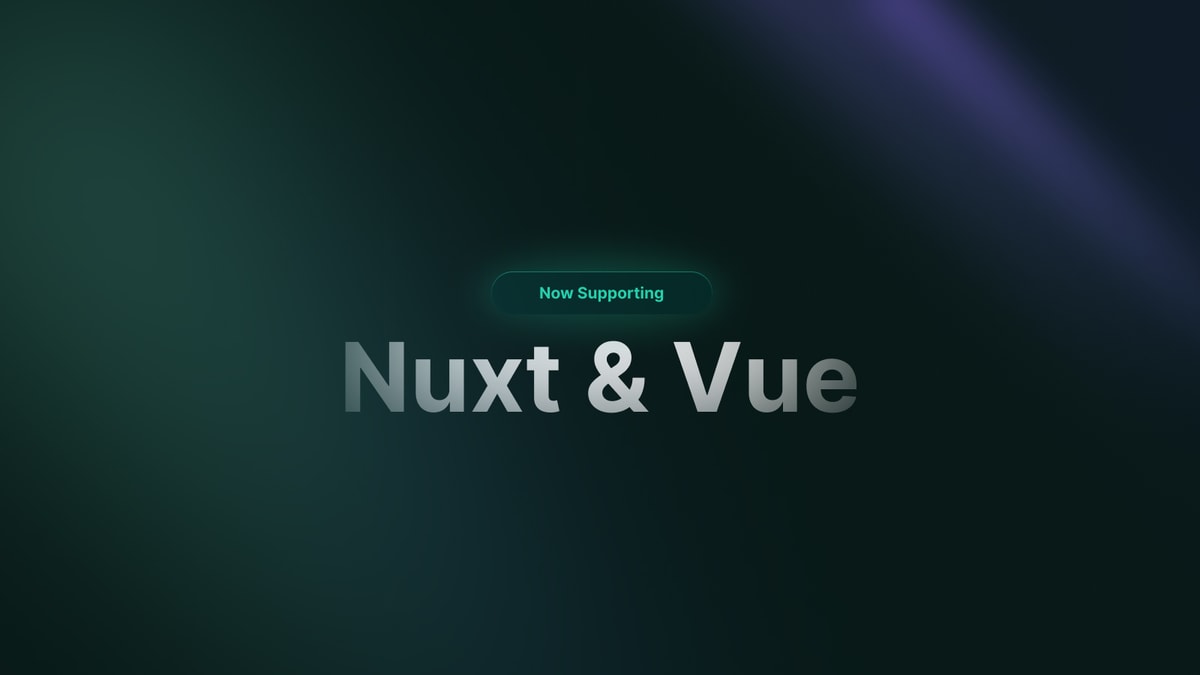
Vue & Nuxt Support, TypeScript Config & Directory Excludes
This week we wanted to expand the horizons of Flytrap's capabilities. As such, this week we are releasing Vue & Nuxt support, with support for SvelteKit to follow next week!Here's what we shipped this week:
Nuxt & Vue supportNow you can integrate your favorite bugcatching tool to your Vue & Nuxt projects! Read our installation Docs for Nuxt or check out our example project here.
Note, that while capturing works like butter, replay for Vue, Nuxt (& Svelte and SvelteKit) are still being worked on, as these frameworks don't explicitly handle state the way that React does, so make sure to stay tuned!TypeScript ConfigOn the Quality-of-Life side of things, we migrated to `c12` to improve our config loading, and allow for using TypeScript to define your Flytrap configuration for that sweet IntelliSense.Directory ExcludesAnother Quality-of-Life improvement we added was the possibility to disable Flytrap for certain directories in the codebase. This allows you to for instance disable directories with stateless UI components, so your captures don't include "useless" context from those components.
Here's how we would ignore a directory for UI components:
import { defineFlytrapConfig } from "useflytrap"export default defineFlytrapConfig({projectId: 'project-id',publicApiKey: 'blah blah',secretApiKey: '*****',privateKey: '*****',mode: 'capture',excludeDirectories: ['./src/components/ui']})
Improvements and fixes
May 19, 2023
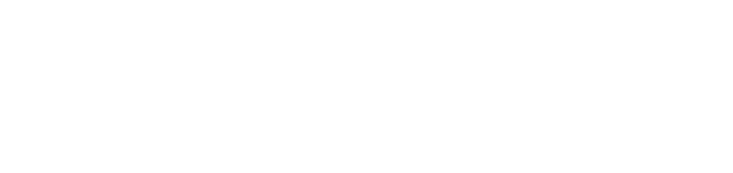
Capture Visualization Revamp, Bundle Size Reduction
Another Friday, another changelog! This week the team has been hard at work with making large foundational changes, that will facilitate many features in the future.Here's what we shipped this week:
Capture Visualization RevampWhen a bug is caught by Flytrap, it's often difficult to get an understanding of what happened leading up to the bug.
Today we're excited to bring you an update that brings a massive improvement to that experience. With the new capture visualization, functions and function calls are visualized as code, which allows you to get an easy understanding of what happened, and which lines were executed. Click on any of the lines to show additional context.Bundle Size ReductionAnother thing we've been hard at work with is reducing the size of transformed code. A large refactoring of the code transform and the introduction of Artifacts API allows us to eliminate about ~70% of code added by the transform.Package IgnoresLike the famous "one last thing" β this one isn't by any means the least. Package ignores allows you to ignore the transformation of any package that you want. This is useful for libraries such as `Zod`, where there's a lot of chained functions, but tracking them adds no useful insight. Also, if using the `next/font` package with NextJS, it can now be ignored to keep NextJS happyπ€.
Here's how we would ignore `Zod` and `next/font` packages:
import { defineFlytrapConfig } from "useflytrap"export default defineFlytrapConfig({projectId: 'project-id',publicApiKey: 'blah blah',secretApiKey: '*****',privateKey: '*****',mode: 'capture',packageIgnores: ['next/font', 'zod']})
Improvements and fixes